Tutorial 자습서 2 - square.js : 시작
페이지 정보
본문
Reactjs.org 자습서 Tutorial 활용 - 순서대로
https://ko.reactjs.org/tutorial/tutorial.html
- 틱택토 Tic Tac Toe 게임 만들기로 자습하기 -
-----------------------
자습서 2 - square.js : 시작
-----------------------
./test/src 폴더에 square.js 파일 생성 : Tic Tac Toe 게임을 작성할 파일.
* square.js 파일에 다음의 초기 코드 입력후 저장
import React from 'react';
import ReactDOM from 'react-dom';
import './square.css'
class Square extends React.Component {
render() {
return (
<button className="square">
{/* TODO */}
</button>
);
}
}
class Board extends React.Component {
renderSquare(i) {
return <Square />;
}
render() {
const status = 'Next player: X';
return (
<div>
<div className="status">{status}</div>
<div className="board-row">
{this.renderSquare(0)}
{this.renderSquare(1)}
{this.renderSquare(2)}
</div>
<div className="board-row">
{this.renderSquare(3)}
{this.renderSquare(4)}
{this.renderSquare(5)}
</div>
<div className="board-row">
{this.renderSquare(6)}
{this.renderSquare(7)}
{this.renderSquare(8)}
</div>
</div>
);
}
}
class Game extends React.Component {
render() {
return (
<div className="game">
<div className="game-board">
<Board />
</div>
<div className="game-info">
<div>{/* status */}</div>
<ol>{/* TODO */}</ol>
</div>
</div>
);
}
}
// ========================================
ReactDOM.render(
<Game />,
document.getElementById('root')
);
export default Square;
연동파일 (3개) - 이 세개의 파일은 꼭 필요하며 튜토리얼 종료시까지 수정할 필요가 없다.
[ square.css, index.js, index.html ]
* square.css 파일 ./test/src 폴더에 square.css 파일 생성 >> 다음의 코드 입력후 저장.
body {
font: 14px "Century Gothic", Futura, sans-serif;
margin: 20px;
}
ol, ul {
padding-left: 30px;
}
.board-row:after {
clear: both;
content: "";
display: table;
}
.status {
margin-bottom: 10px;
}
.square {
background: #fff;
border: 1px solid #999;
float: left;
font-size: 24px;
font-weight: bold;
line-height: 34px;
height: 34px;
margin-right: -1px;
margin-top: -1px;
padding: 0;
text-align: center;
width: 34px;
}
.square:focus {
outline: none;
}
.kbd-navigation .square:focus {
background: #ddd;
}
.game {
display: flex;
flex-direction: row;
}
.game-info {
margin-left: 20px;
}
* index.js 파일 ./test/src 폴더에 생성되어 있음.
index.js 파일 내용 변경
- import App from './App'; 를 import Square from './square'; 로
- <App />를 <Square />로 ...
일부만 수정후 저장.
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import Square from './square';
import reportWebVitals from './reportWebVitals';
ReactDOM.render(
<React.StrictMode>
<Square />
</React.StrictMode>,
document.getElementById('root')
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
* index.html 파일 : 터미널에서 npx create-react-app으로 프로젝트를 생성하면 자동으로 생성되는 기본 파일들 중 하나이며 일반적으로 변경할 필요가 없으므로 그대로 사용한다.
----------
터미널에서 npm start 로 개발 서버 실행.
초기 코드 서버 실행결과 캡쳐화면 (웹브라우저)
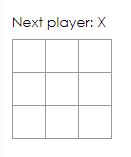